Excel 数据修复:插入缺失时间并使用 EM 算法填充数据
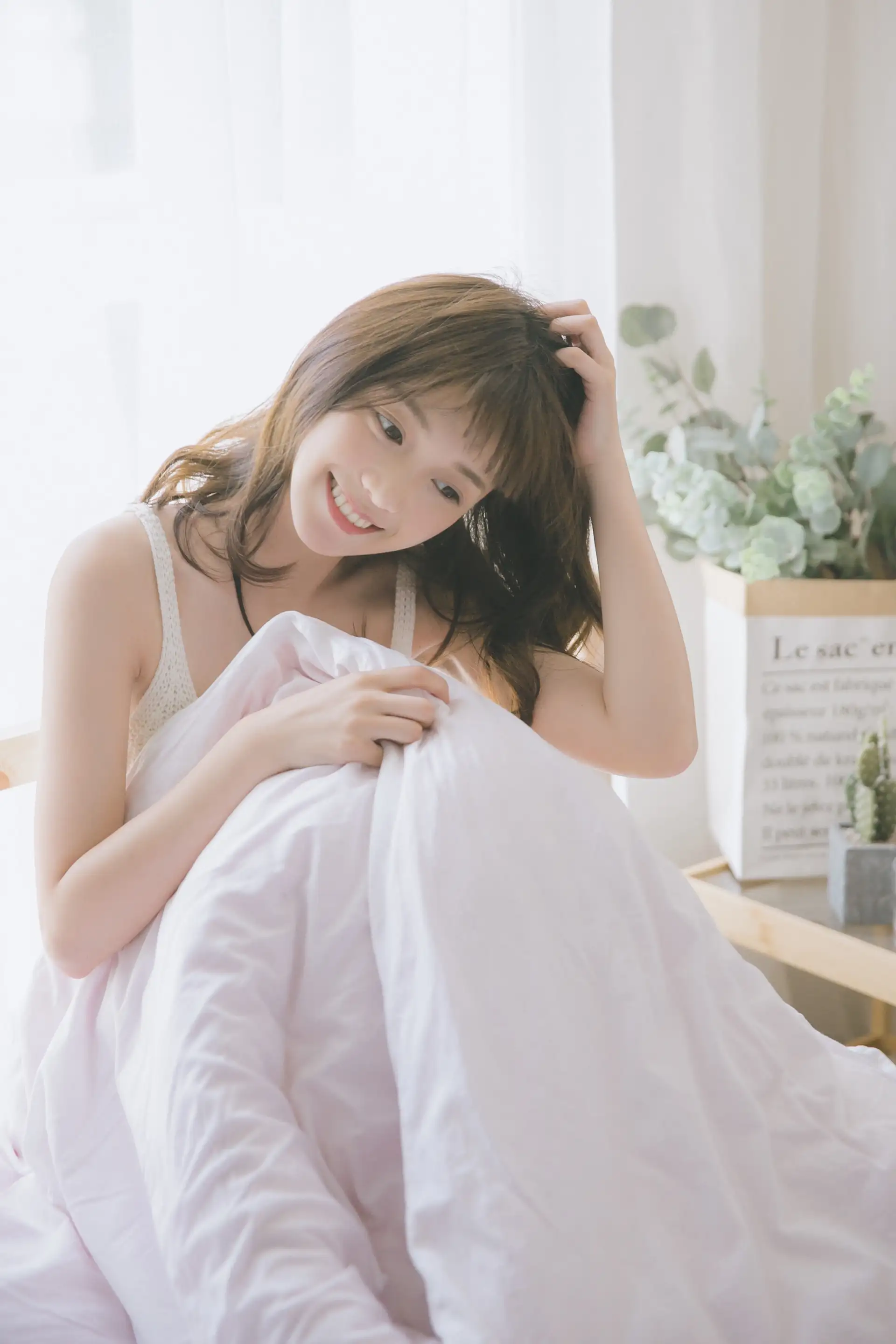
import org.apache.poi.ss.usermodel.*;
import java.io.FileInputStream; import java.io.FileOutputStream; import java.text.DecimalFormat; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.ArrayList; import java.util.Date; import java.util.List; import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class EM01 {
public static void main(String[] args) {
// 定义输入文件和输出文件的路径
String inputFile = "input.xlsx";
try (Workbook workbook = WorkbookFactory.create(new FileInputStream(inputFile))) { // 使用工作簿工厂创建 Excel 工作簿
Sheet sheet = workbook.getSheet("P1"); // 获取 P1 工作表
DecimalFormat df = new DecimalFormat("#.##"); // 创建 Decimal 格式化器,用于保留两位小数
// 创建新的工作表
String newSheetName = "P1-1";
int sheetIndex = workbook.getSheetIndex(newSheetName);
Sheet newDataSheet;
if (sheetIndex >= 0) {
workbook.removeSheetAt(sheetIndex);
}
newDataSheet = workbook.createSheet(newSheetName);
// 复制原工作表的数据到新的工作表
for (int i = 0; i <= sheet.getLastRowNum(); i++) {
Row oldRow = sheet.getRow(i);
Row newRow = newDataSheet.createRow(i);
if (oldRow != null) {
for (int j = 0; j < oldRow.getLastCellNum(); j++) {
Cell oldCell = oldRow.getCell(j);
Cell newCell = newRow.createCell(j);
if (oldCell != null) {
if(oldCell.getCellType() == CellType.STRING){
newCell.setCellValue(oldCell.getStringCellValue());
} else if(oldCell.getCellType() == CellType.NUMERIC){
newCell.setCellValue(oldCell.getNumericCellValue());
} else if(oldCell.getCellType() == CellType.BOOLEAN){
newCell.setCellValue(oldCell.getBooleanCellValue());
} else if(oldCell.getCellType() == CellType.FORMULA){
newCell.setCellValue(oldCell.getCellFormula());
} else if(oldCell.getCellType() == CellType.ERROR){
newCell.setCellValue(oldCell.getErrorCellValue());
}
// 复制原单元格的样式到新单元格
CellStyle oldCellStyle = oldCell.getCellStyle();
CellStyle newCellStyle = workbook.createCellStyle();
newCellStyle.cloneStyleFrom(oldCellStyle);
newCell.setCellStyle(newCellStyle);
}
}
}
}
// 对每一行进行处理
for (int i = 1; i <= newDataSheet.getLastRowNum(); i++) {
Row row = newDataSheet.getRow(i); // 获取行对象
if (row != null) {
Cell cell = row.getCell(1); // 获取第二列单元格
if (cell == null || cell.getCellType() == CellType.BLANK) { // 判断单元格是否为空或者空白
double avg = calculateEM(newDataSheet, i, 1); // 计算EM算法填充的值
if (avg > 0) { // 如果填充的值大于 0
cell = row.createCell(1); // 创建新的单元格
cell.setCellValue(Double.parseDouble(df.format(avg))); // 将填充的值填入单元格
// 设置单元格的数据类型为数值类型
CellStyle cellStyle = workbook.createCellStyle();
DataFormat dataFormat = workbook.createDataFormat();
cellStyle.setDataFormat(dataFormat.getFormat("0.00"));
cell.setCellStyle(cellStyle);
}
}
}
}
// 插入缺失的时间和数据
insertMissingData(newDataSheet);
// 将工作簿写入输入文件
FileOutputStream outputStream = new FileOutputStream(inputFile);
workbook.write(outputStream);
outputStream.close();
System.out.println("Data filling completed."); // 输出信息
} catch (Exception e) { // 捕获异常
e.printStackTrace();
}
}
// 计算EM算法填充的值
private static double calculateEM(Sheet sheet, int rowIndex, int columnIndex) {
Row row;
double missingValue = 0; // 缺失值
row = sheet.getRow(rowIndex); // 获取当前行对象
if (row != null) {
Cell cell = row.getCell(columnIndex); // 获取指定列的单元格
if (cell != null && cell.getCellType() == CellType.NUMERIC) {
missingValue = cell.getNumericCellValue(); // 缺失值为单元格中的值
} else if (cell != null && cell.getCellType() == CellType.STRING) {
try {
missingValue = Double.parseDouble(cell.getStringCellValue()); // 转换为数字类型
} catch (NumberFormatException e) {
missingValue = 0; // 转换失败则缺失值为 0
}
}
}
List<Double> data = new ArrayList<>(); // 存储数据
for (int i = 0; i <= sheet.getLastRowNum(); i++) { // 对每一行进行处理
row = sheet.getRow(i); // 获取行对象
if (row != null) {
Cell cell = row.getCell(columnIndex); // 获取指定列的单元格
if (cell != null && cell.getCellType() == CellType.NUMERIC) {
data.add(cell.getNumericCellValue()); // 将数据添加到列表中
} else if (cell != null && cell.getCellType() == CellType.STRING) {
try {
double value = Double.parseDouble(cell.getStringCellValue()); // 转换为数字类型
data.add(value); // 将数据添加到列表中
} catch (NumberFormatException e) {
// 转换失败,忽略该值
}
}
}
}
if (data.size() > 0 && missingValue >= 0) { // 如果存在数据且缺失值大于 0
double sum = 0; // 总和
int count = 0; // 非缺失值个数
for (double value : data) { // 遍历数据
if (value >= 0) { // 非缺失值
sum += value; // 累加非缺失值
count++; // 非缺失值个数加一
}
}
if (count > 0) { // 如果存在非缺失值
double average = sum / count; // 计算平均值
double variance = 0; // 方差
for (double value : data) { // 遍历数据
if (value >= 0) { // 非缺失值
variance += Math.pow(value - average, 2); // 累加方差
}
}
variance /= count; // 计算方差
double stdDeviation = Math.sqrt(variance); // 标准差
double filledValue = generateRandomValue(average, stdDeviation); // 生成符合正态分布的随机值
return filledValue; // 返回填充的值
}
}
return 0; // 如果不存在数据或缺失值小于等于0,返回 0
}
// 生成符合正态分布的随机值
private static double generateRandomValue(double average, double stdDeviation) {
double value;
do {
value = average + stdDeviation * Math.random(); // 生成随机值
} while (value < 0); // 保证生成的值大于等于 0
return value;
}
// 插入缺失的时间和数据
private static void insertMissingData(Sheet sheet) {
Workbook workbook = sheet.getWorkbook(); // 获取工作簿对象
for (int i = 1; i < sheet.getLastRowNum(); i++) {
Row currentRow = sheet.getRow(i);
Row nextRow = sheet.getRow(i + 1);
if (currentRow != null && nextRow != null) {
Cell currentDateCell = currentRow.getCell(0);
Cell nextDateCell = nextRow.getCell(0);
if (currentDateCell != null && nextDateCell != null && currentDateCell.getCellType() == CellType.NUMERIC && nextDateCell.getCellType() == CellType.NUMERIC) {
Date currentDate = currentDateCell.getDateCellValue();
Date nextDate = nextDateCell.getDateCellValue();
long diff = nextDate.getTime() - currentDate.getTime();
long diffDays = diff / (24 * 60 * 60 * 1000);
while (diffDays > 10) { // 循环插入时间,直到时间间隔不超过10天
int insertCount = (int) (diffDays / 10);
for (int j = 1; j <= insertCount; j++) {
Row newRow = sheet.createRow(i + j); // 插入新行
Cell newDateCell = newRow.createCell(0);
Cell newDataCell = newRow.createCell(1);
Date insertDate = new Date(currentDate.getTime() + j * 10 * 24 * 60 * 60 * 1000); // 计算插入时间
newDateCell.setCellValue(insertDate);
// 设置新单元格的格式与原单元格一致
CellStyle oldDateCellStyle = currentDateCell.getCellStyle();
CellStyle newDateCellStyle = workbook.createCellStyle();
newDateCellStyle.cloneStyleFrom(oldDateCellStyle);
newDateCell.setCellStyle(newDateCellStyle);
// 设置新数据单元格的格式与原单元格一致
CellStyle oldDataCellStyle = currentRow.getCell(1).getCellStyle();
CellStyle newDataCellStyle = workbook.createCellStyle();
newDataCellStyle.cloneStyleFrom(oldDataCellStyle);
newDataCell.setCellStyle(newDataCellStyle);
newDataCell.setCellValue(calculateEM(sheet, i, 1)); // 使用EM算法填充数据
}
i += insertCount; // 更新行索引
currentDate = insertDate; // 更新当前时间
nextDate = sheet.getRow(i + 1).getCell(0).getDateCellValue(); // 更新下一个时间
diff = nextDate.getTime() - currentDate.getTime();
diffDays = diff / (24 * 60 * 60 * 1000);
}
}
}
}
}
// 解析日期
private static Date parseDate(String dateString) {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy/MM/dd HH:mm"); // 创建日期格式化器
try {
return dateFormat.parse(dateString); // 解析日期
} catch (ParseException e) { // 捕获异常
e.printStackTrace();
return null; // 返回 null
}
}
}
原文地址: https://cveoy.top/t/topic/fP7J 著作权归作者所有。请勿转载和采集!